CHAPTER 7
Quantum Fractal Theory
In quantum fractal theory, the entire Mandelbrot plane is mapped by calculating the state change (△k) in both the x-direction and the y-direction. The state change for each direction is calculated separately.
For any Mandelbrot in Cartesian coordinates, a count, k is incremented when f(x, y) > escape.
In this case, (x²-y²)² + (2.0*x*y)² > 6.25
(xs, ys) = (i/scale, j/scale) where (i, j) is the pixel location.
To obtain the complete map for each pixel (i, j):
i is held constant while j is incremented for kⱼ, j is held constant while i is incremented for kᵢ
or
j is held constant while i is incremented for kᵢ, i is held constant while j is incremented for kⱼ
For every point, (x,y) at pixel (i,j), there are two associated △k: kⱼ and kᵢ.
△kⱼ = kⱼ - kj-1 for the y-direction.
△kᵢ = kᵢ - ki-1 for the x-direction.
If △kⱼ≠0, the pixel is plotted in the y-direction.
If △kᵢ≠0, the pixel is plotted in the x-direction.
k is always positive; △k is positive, negative, or zero.
For the graphs to the right, both △kⱼ≠0 and △kᵢ≠0 are plotted on the same graph.
The first graph shows △kⱼ≠0 followed by △kᵢ≠0.
The second graph shows △kᵢ≠0 followed by △kⱼ≠0.
△kⱼ≠0 and △kᵢ≠0 contain some pixels (i, j) in common.
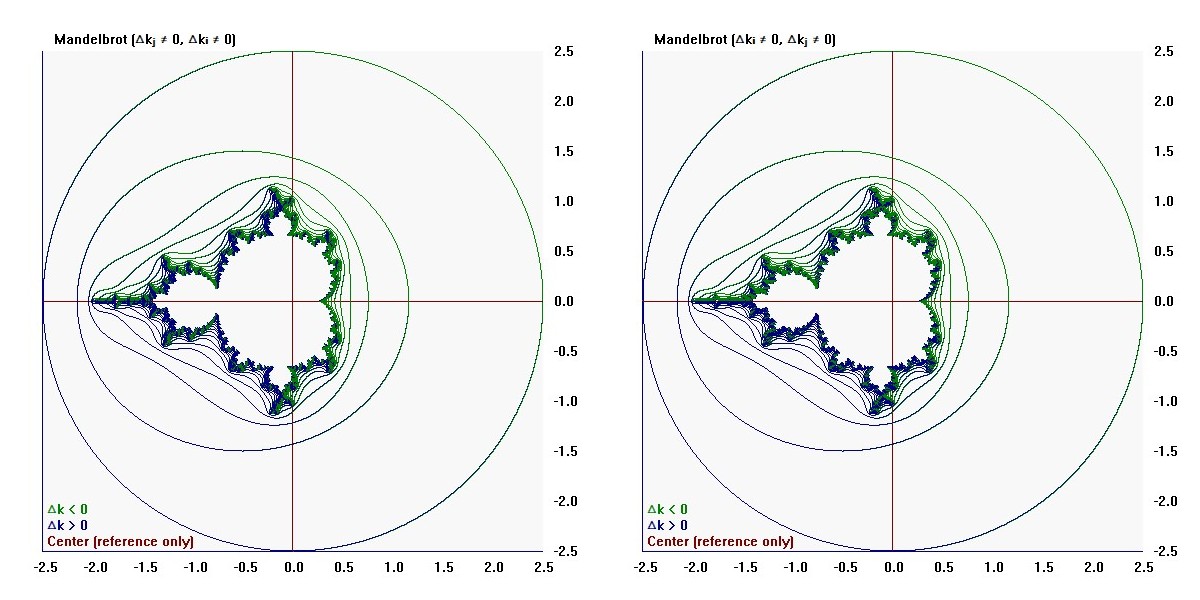
Here is pseudocode for the Mandelbrot set where (x² - y², 2.0*x*y) is the build. In the first loop, x remains constant while △k = △kⱼ in the y-direction is calculated. Each start pixel(i, j) is plotted when △kⱼ ≠ 0. In the second loop, y remains constant while △k = △kᵢ in the x-direction is calculated. Each start pixel(i, j) is plotted when △kᵢ ≠ 0. If there are points in common (and there are) for △kⱼ and △kᵢ, the points for △kᵢ in the second loop will overlay any points for △kⱼ in the first loop.
for (int i = -250; i ≤ 250; i++) { oldk = 0; for (int j = -250; j ≤ 250; j++) { x = 0.0; y = 0.0; xs = i / 100.0; ys = j / 100.0; k = 0; do { k = k + 1; xnew = x * x - y * y + xs; ynew = 2.0 * x * y + ys; x = xnew; y = ynew; } while ((k ≤ 24) && (x * x + y * y ≤ 6.25)); if (k != oldk) PlotPixel(i, j, color)); oldk = k; } } for (int j = -250; j ≤ 250; j++) { oldk = 0; for (int i = -250; i ≤ 250; i++) { x = 0.0; y = 0.0; xs = i / 100.0; ys = j / 100.0; k = 0; do { k = k + 1; xnew = x * x - y * y + xs; ynew = 2.0 * x * y + ys; x = xnew; y = ynew; } while ((k ≤ 24) && (x * x + y * y ≤ 6.25)); if (k != oldk) PlotPixel(i, j, color); oldk = k; } }
Here is pseudocode for the Mandelbrot set where (x² - y², 2.0*x*y) is the build. In the first loop, y remains constant while △k = △kᵢ in the x-direction is calculated. Each start pixel(i, j) is plotted when △kᵢ ≠ 0. In the second loop, x remains constant while △k = △kⱼ in the y-direction is calculated. Each start pixel(i, j) is plotted when △kⱼ ≠ 0. If there are points in common (and there are) for △kᵢ and △kⱼ, the points for △kⱼ in the second loop will overlay any points for △kᵢ in the first loop.
for (int j = -250; j ≤ 250; j++) { oldk = 0; for (int i = -250; i ≤ 250; i++) { x = 0.0; y = 0.0; xs = i / 100.0; ys = j / 100.0; k = 0; do { k = k + 1; xnew = x * x - y * y + xs; ynew = 2.0 * x * y + ys; x = xnew; y = ynew; } while ((k ≤ 24) && (x * x + y * y ≤ 6.25)); if (k != oldk) PlotPixel(i, j, color); oldk = k; } } for (int i = -250; i ≤ 250; i++) { oldk = 0; for (int j = -250; j ≤ 250; j++) { x = 0.0; y = 0.0; xs = i / 100.0; ys = j / 100.0; k = 0; do { k = k + 1; xnew = x * x - y * y + xs; ynew = 2.0 * x * y + ys; x = xnew; y = ynew; } while ((k ≤ 24) && (x * x + y * y ≤ 6.25)); if (k != oldk) PlotPixel(i, j, color); oldk = k; } }